Step1) Create a ASP.NET Empty WebSite using C# 4.0/4.5
Step 2) Add new Page name it as Default.aspx
Step3) Add DropDownList and Label
add event handler for OnSelectedIndexChanged and Autopostback set to true.otheriwise post back will not occur when selection changes in DropDownList
<asp:DropDownList ID="DropDownList1" runat="server" AutoPostBack="true" OnSelectedIndexChanged="DropDownList1_SelectedIndexChanged">
<asp:ListItem Selected="True">Select Logical Drive</asp:ListItem>
</asp:DropDownList>
<br />
<br />
<asp:Label ID="lblMsg" runat="server" Text="Label"></asp:Label>
Step 4) Add a Method to get LogicalDrives
String[] GetLogicalDrives()
{
return System.Environment.GetLogicalDrives();
}
Step 5) Add a method to get DriveInfo
String GetDriveInfo(String drive)
{
string str = "Drive not Ready";
try
{
DriveInfo dInfo = new DriveInfo(drive);
if (dInfo.IsReady)
{
str = "<b>AvailableFreeSpace</b>:" +Get_Size_in_KB_MB_GB((ulong)dInfo.AvailableFreeSpace,0) + "<br/>";
str += "<b>TotalFreeSpace</b>:" + Get_Size_in_KB_MB_GB((ulong)dInfo.TotalFreeSpace,0) + "<br/>";
str += "<b>TotalSize</b>:" + Get_Size_in_KB_MB_GB((ulong)dInfo.TotalSize,0) + "<br/>";
str += "<b>VolumeLabel</b>:" + dInfo.VolumeLabel + "<br/>";
str += "<b>RootDirectory</b>:" + dInfo.RootDirectory + "<br/>";
str += "<b>Name</b>:" + dInfo.Name + "<br/>";
str += "<b>DriveType</b>:" + dInfo.DriveType + "<br/>";
str += "<b>DriveFormat</b>:" + dInfo.DriveFormat + "<br/>";
}
}
catch (Exception ex)
{
}
return str;
}
Step 6) Bind Logical Drives Array to DropDownList
DropDownList1.DataSource = GetLogicalDrives();
DropDownList1.DataBind();
Step 7) Add Default value to DropDownList
DropDownList1.Items.Insert(0,"Select Logical Drive");
Step 8) Add Code for DropDownList1_SelectedIndexChanged
ListItem item=DropDownList1.SelectedItem;
lblMsg.Text=GetDriveInfo(item.Text);
Step 9) Convert Bytes to KB,MB,GB in C#
String[] sizeArry = new String[] { "Byes", "KB", "MB", "GB" };
String Get_Size_in_KB_MB_GB(ulong sizebytes, int index)
{
if (sizebytes < 1000) return sizebytes + sizeArry[index];
else return Get_Size_in_KB_MB_GB(sizebytes / 1024, ++index);
}
Step 10) Run the WebPage Default.aspx
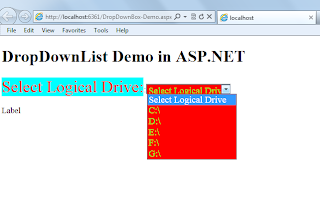
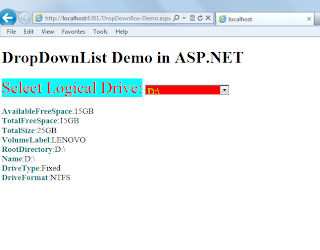
Complete Source Code
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="DropDownBox-Demo.aspx.cs" Inherits="WebApplication1.DropDownBox_Demo" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<style type="text/css">
.auto-style1
{
font-size: xx-large;
color:red;
background-color:aqua;
}
b
{
color:teal;
}
</style>
</head>
<body>
<form id="form1" runat="server">
<div>
<h1>DropDownList Demo in ASP.NET</h1>
<span class="auto-style1">Select Logical Drive:</span>:<asp:DropDownList ID="DropDownList1" runat="server" AutoPostBack="true" Height="21px" OnSelectedIndexChanged="DropDownList1_SelectedIndexChanged" Width="164px" BackColor="Red" Font-Names="Times New Roman" Font-Size="Larger" ForeColor="#66FF33">
<asp:ListItem Selected="True">Select Logical Drive</asp:ListItem>
</asp:DropDownList>
<br />
<br />
<asp:Label ID="lblMsg" runat="server" Text="Label"></asp:Label>
</div>
</form>
</body>
</html>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<style type="text/css">
.auto-style1
{
font-size: xx-large;
color:red;
background-color:aqua;
}
b
{
color:teal;
}
</style>
</head>
<body>
<form id="form1" runat="server">
<div>
<h1>DropDownList Demo in ASP.NET</h1>
<span class="auto-style1">Select Logical Drive:</span>:<asp:DropDownList ID="DropDownList1" runat="server" AutoPostBack="true" Height="21px" OnSelectedIndexChanged="DropDownList1_SelectedIndexChanged" Width="164px" BackColor="Red" Font-Names="Times New Roman" Font-Size="Larger" ForeColor="#66FF33">
<asp:ListItem Selected="True">Select Logical Drive</asp:ListItem>
</asp:DropDownList>
<br />
<br />
<asp:Label ID="lblMsg" runat="server" Text="Label"></asp:Label>
</div>
</form>
</body>
</html>
No comments:
Post a Comment