how to get logical drives and driveinfo in asp.net using C#
Step1) Create a ASP.NET Empty WebSite using VB.NET 4.0/4.5
Step 2) Add new Page name it as Default.aspx
Step3) Add DropDownList and Label
add event handler for OnSelectedIndexChanged and Autopostback set to true.otheriwise post back will not occur when selection changes in DropDownList
<asp:DropDownList ID="DropDownList1" runat="server" AutoPostBack="true" OnSelectedIndexChanged="DropDownList1_SelectedIndexChanged">
<asp:ListItem Selected="True">Select Logical Drive</asp:ListItem>
</asp:DropDownList>
<br />
<br />
<asp:Label ID="lblMsg" runat="server" Text="Label"></asp:Label>
Step 4) Add a Method to get LogicalDrives
String() GetLogicalDrives()
{
Return System.Environment.GetLogicalDrives()
}
Step 5) Add a method to get DriveInfo
Private Function GetDriveInfo(drive As [String]) As [String]
Dim str As String = "Drive not Ready"
Try
Dim dInfo As New DriveInfo(drive)
If dInfo.IsReady Then
str = "<b>AvailableFreeSpace</b>:" + Get_Size_in_KB_MB_GB(CULng(dInfo.AvailableFreeSpace), 0) + "<br/>"
str += "<b>TotalFreeSpace</b>:" + Get_Size_in_KB_MB_GB(CULng(dInfo.TotalFreeSpace), 0) + "<br/>"
str += "<b>TotalSize</b>:" + Get_Size_in_KB_MB_GB(CULng(dInfo.TotalSize), 0) + "<br/>"
str += "<b>VolumeLabel</b>:" + dInfo.VolumeLabel + "<br/>"
str += "<b>RootDirectory</b>:" + dInfo.RootDirectory + "<br/>"
str += "<b>Name</b>:" + dInfo.Name + "<br/>"
str += "<b>DriveType</b>:" + dInfo.DriveType + "<br/>"
str += "<b>DriveFormat</b>:" + dInfo.DriveFormat + "<br/>"
End If
Catch ex As Exception
End Try
Return str
End Function
Private Function GetDriveInfo(drive As [String]) As [String]
Dim str As String = "Drive not Ready"
Try
Dim dInfo As New DriveInfo(drive)
If dInfo.IsReady Then
str = "<b>AvailableFreeSpace</b>:" + Get_Size_in_KB_MB_GB(CULng(dInfo.AvailableFreeSpace), 0) + "<br/>"
str += "<b>TotalFreeSpace</b>:" + Get_Size_in_KB_MB_GB(CULng(dInfo.TotalFreeSpace), 0) + "<br/>"
str += "<b>TotalSize</b>:" + Get_Size_in_KB_MB_GB(CULng(dInfo.TotalSize), 0) + "<br/>"
str += "<b>VolumeLabel</b>:" + dInfo.VolumeLabel + "<br/>"
str += "<b>RootDirectory</b>:" + dInfo.RootDirectory + "<br/>"
str += "<b>Name</b>:" + dInfo.Name + "<br/>"
str += "<b>DriveType</b>:" + dInfo.DriveType + "<br/>"
str += "<b>DriveFormat</b>:" + dInfo.DriveFormat + "<br/>"
End If
Catch ex As Exception
End Try
Return str
End Function
Step 6) Bind Logical Drives Array to DropDownList
DropDownList1.DataSource = GetLogicalDrives()
DropDownList1.DataBind()
Step 7) Add Default value to DropDownList
DropDownList1.Items.Insert(0,"Select Logical Drive")
Step 8) Add Code for DropDownList1_SelectedIndexChanged
ListItem item=DropDownList1.SelectedItem
lblMsg.Text=GetDriveInfo(item.Text)
Step 9) Convert Bytes to KB,MB,GB in C#
Private sizeArry As [String]() = New [String]() {"Byes", "KB", "MB", "GB"}
Private Function Get_Size_in_KB_MB_GB(sizebytes As ULong, index As Integer) As [String]
If sizebytes < 1000 Then
Return sizebytes + sizeArry(index)
Else
Return Get_Size_in_KB_MB_GB(sizebytes / 1024, System.Threading.Interlocked.Increment(index))
End If
End Function
Private Function Get_Size_in_KB_MB_GB(sizebytes As ULong, index As Integer) As [String]
If sizebytes < 1000 Then
Return sizebytes + sizeArry(index)
Else
Return Get_Size_in_KB_MB_GB(sizebytes / 1024, System.Threading.Interlocked.Increment(index))
End If
End Function
Step 10) Run the WebPage Default.aspx
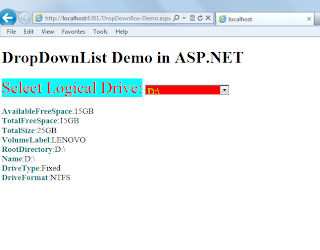
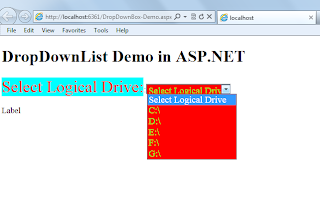
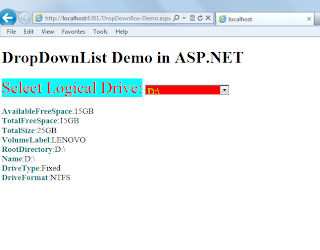
Complete Source Code
Default.aspx
<%@
Page Language="VB" AutoEventWireup="true"
CodeBehind="DropDownBox-Demo.aspx.VB"
Inherits="WebApplication1.DropDownBox_Demo" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<style type="text/css">
.auto-style1
{
font-size: xx-large;
color:red;
background-color:aqua;
}
b
{
color:teal;
}
</style>
</head>
<body>
<form id="form1" runat="server">
<div>
<h1>DropDownList Demo in ASP.NET</h1>
<span class="auto-style1">Select Logical Drive:</span>:<asp:DropDownList ID="DropDownList1" runat="server" AutoPostBack="true" Height="21px" OnSelectedIndexChanged="DropDownList1_SelectedIndexChanged" Width="164px" BackColor="Red" Font-Names="Times New Roman" Font-Size="Larger" ForeColor="#66FF33">
<asp:ListItem Selected="True">Select Logical Drive</asp:ListItem>
</asp:DropDownList>
<br />
<br />
<asp:Label ID="lblMsg" runat="server" Text="Label"></asp:Label>
</div>
</form>
</body>
</html>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<style type="text/css">
.auto-style1
{
font-size: xx-large;
color:red;
background-color:aqua;
}
b
{
color:teal;
}
</style>
</head>
<body>
<form id="form1" runat="server">
<div>
<h1>DropDownList Demo in ASP.NET</h1>
<span class="auto-style1">Select Logical Drive:</span>:<asp:DropDownList ID="DropDownList1" runat="server" AutoPostBack="true" Height="21px" OnSelectedIndexChanged="DropDownList1_SelectedIndexChanged" Width="164px" BackColor="Red" Font-Names="Times New Roman" Font-Size="Larger" ForeColor="#66FF33">
<asp:ListItem Selected="True">Select Logical Drive</asp:ListItem>
</asp:DropDownList>
<br />
<br />
<asp:Label ID="lblMsg" runat="server" Text="Label"></asp:Label>
</div>
</form>
</body>
</html>
Default.aspx.vb
Imports System
Imports System.Collections.Generic
Imports System.Linq
Imports System.Web
Imports System.Web.UI
Imports System.Web.UI.WebControls
Imports System.IO
Namespace WebApplication1
Public Partial Class DropDownBox_Demo
Inherits System.Web.UI.Page
Protected Sub Page_Load(sender As Object, e As EventArgs)
If Not IsPostBack Then
DropDownList1.DataSource = GetLogicalDrives()
DropDownList1.DataBind()
DropDownList1.Items.Insert(0, "Select Logical Drive")
End If
End Sub
Protected Sub DropDownList1_SelectedIndexChanged(sender As Object, e As EventArgs)
Dim item As ListItem = DropDownList1.SelectedItem
lblMsg.Text = GetDriveInfo(item.Text)
End Sub
Private Function GetLogicalDrives() As [String]()
Return System.Environment.GetLogicalDrives()
End Function
Private Function GetDriveInfo(drive As [String]) As [String]
Dim str As String = "Drive not Ready"
Try
Dim dInfo As New DriveInfo(drive)
If dInfo.IsReady Then
str = "<b>AvailableFreeSpace</b>:" + Get_Size_in_KB_MB_GB(CULng(dInfo.AvailableFreeSpace), 0) + "<br/>"
str += "<b>TotalFreeSpace</b>:" + Get_Size_in_KB_MB_GB(CULng(dInfo.TotalFreeSpace), 0) + "<br/>"
str += "<b>TotalSize</b>:" + Get_Size_in_KB_MB_GB(CULng(dInfo.TotalSize), 0) + "<br/>"
str += "<b>VolumeLabel</b>:" + dInfo.VolumeLabel + "<br/>"
str += "<b>RootDirectory</b>:" + dInfo.RootDirectory + "<br/>"
str += "<b>Name</b>:" + dInfo.Name + "<br/>"
str += "<b>DriveType</b>:" + dInfo.DriveType + "<br/>"
str += "<b>DriveFormat</b>:" + dInfo.DriveFormat + "<br/>"
End If
Catch ex As Exception
End Try
Return str
End Function
Private Function Get_Size_in_KB_MB_GB(sizebytes As ULong, index As Integer) As [String]
If sizebytes < 1000 Then
Return sizebytes + sizeArry(index)
Else
Return Get_Size_in_KB_MB_GB(sizebytes / 1024, System.Threading.Interlocked.Increment(index))
End If
End Function
Private sizeArry As [String]() = New [String]() {"Byes", "KB", "MB", "GB"}
End Class
End Namespace
No comments:
Post a Comment